Integrating with Existing Code
A core tenet of Flyde is that it should integrate with existing code, and not replace it.
To achieve this, Flyde provides a runtime library that allows you to load and run .flyde files, and a webpack loader that allows you to load .flyde files directly from your code. Also, custom nodes can be implemented using TypeScript or JavaScript (more on that in the custom nodes article).
For example, given a .flyde flow that converts Celsius to Fahrenheit:
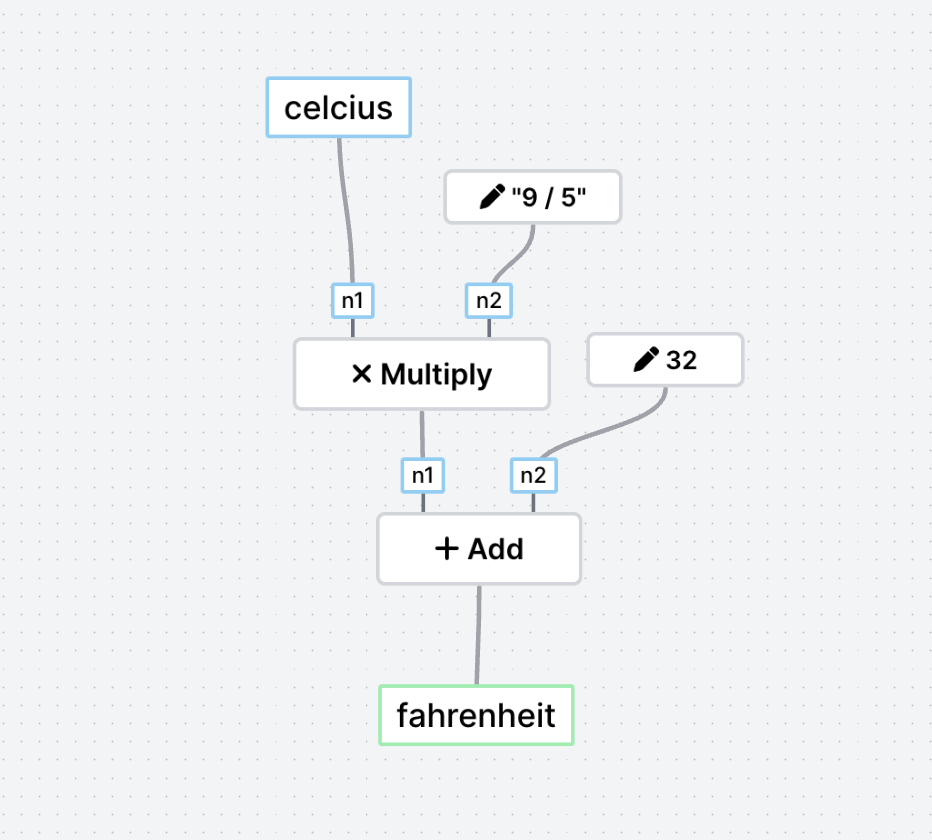
You can load and run it from your code as following:
import { loadFlow } from "@flyde/runtime";
const execute = await loadFlow("./celsius-to-fahrenheit.flyde");
const inputs = { celsius: 0 }; // "celcius" is a main input in the flow, therefore it must be provided when executing the flow
const { result } = execute(inputs); // execute returns a "result" promise, along with a cleanup function that can be used to cancel the execution.
const { fahrenheit } = await result; // each output in the flow is a property on the result object
console.log(result.fahrenheit); // 32
The execute
function returns an object with a result
property - a promise that resolves to the result of the flow.
You may also listen to outputs before the flow completes by passing an "onOutputs" callback to the second argument of execute
:
const { result } = execute(inputs, {
onOutputs: (key, value) => {
console.log(`output with key ${key} emitted value ${value}`);
},
});
The example above assumes a Node.js environment. Loading Flyde in a browser environment is possible, but not yet documented. Checkout the website's source code, here and here for an example of how to do it.
Learn more about the lifecycle of a flow in the advanced concepts article.
Another key method of intgrating with existing code is by creating your own custom nodes. Learn more about that in the custom nodes article.